NiTi multilayer example#
For our first example, we will consider a simple hard matter system, where there is a repeating multilayer of nickel and titanium that has been deposited on a piece of silicon. The process by which these systems are prepared typically involves the disposition of a layer of titanium, followed by a layer of nickel, followed by titanium, this process is repeated until the desired number of layers is reached. Below we show, graphically, a model that we may use for the simulation of reflectometry data from such a system.
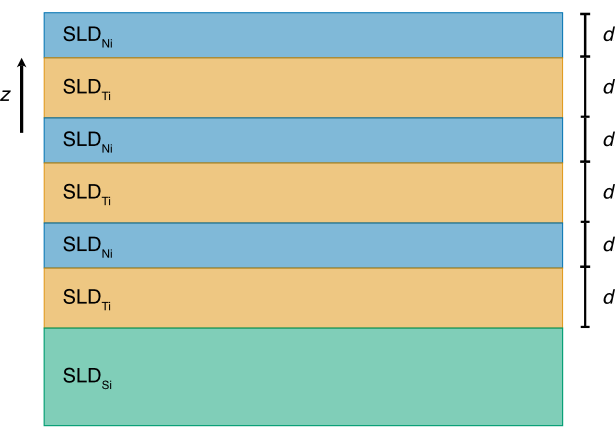
Fig. 7 A graphical example of the NiTi multilayer system consisting of three repeats.#
A common assumption that is made in the analysis of reflectometry data from such a sample include that the structure of each repeat is the same. Therefore the model can be defined such that there are only a limited number of variables. However, this can still be unclear, as three of these parameters will be the scattering length densities of nickel, titanium, and silicon. While these values can be obtained from online databases, we can also reparameterise these in terms of the mass density of the material.
Scattering length density → Mass density#
The scattering length density, \(\textrm{SLD}\), for a material is directly related to its mass density, \(\rho\),
where, \(N_a\) is Avogadro’s constant, \(b_i\) are the neutron scattering lengths for each of the \(N\) elements in the material, and \(M_w\) is the molecular mass of the material. Therefore, if we consider nickel, which has a density of \(8.908\) gcm-3 at room temperature and a scattering length of \(10.3\) fm, the scattering length density is found as follows,
We evaluate this in the code below.
import numpy as np
import pint
from scipy.constants import N_A
u = pint.UnitRegistry()
N_A /= u.mol
def rho_to_SLD(rho: float, b: np.ndarray, m_w: float) -> float:
"""
:param rho: mass density
:param b: neutron scattering length for individual atoms
:param m_w: molecular weight
"""
return rho * N_A * np.sum(b) / (m_w)
rho_Ni = 8.91 * u.g / (u.cm ** 3)
b_Ni = np.array([10.3]) * u.fm
m_w_Ni = 58.69 * u.g / u.mol
SLD_Ni = rho_to_SLD(rho_Ni, b_Ni, m_w_Ni)
print(f'SLD_Ni = {SLD_Ni.to(1 / (u.angstrom ** 2)):.3e}')
SLD_Ni = 9.417e-06 / angstrom ** 2
Using this approach, the parameter that we vary in the model-dependent analysis approach can be the mass density rather than the more abstract scattering length density. Additionally, this means that we can more easily constrain our fitting process, by assigning a set of bounds on our parameter (more on this later) based on reasonable values for the density of the material. The majority of fitting packages can allow the reflectometry analysis model to be defined in terms of the parameters such as mass density, instead of the more traditional scattering length density.
We can also find the scattering length density of titanium by the same approach as above.
rho_Ti = 4.51 * u.g / (u.cm ** 3)
b_Ti = np.array([-3.438]) * u.fm
m_w_Ti = 47.867 * u.g / u.mol
SLD_Ti = rho_to_SLD(rho_Ti, b_Ti, m_w_Ti)
print(f'SLD_Ti = {SLD_Ti.to(1 / (u.angstrom ** 2)):.3e}')
SLD_Ti = -1.951e-06 / angstrom ** 2